How to: Create Custom Notifications in Content Hub
Have you ever wondered how to send custom notifications in Sitecore Content Hub? Perhaps you want to send a notification to all users every time a new image containing a cat is uploaded. (We won't judge. 😻). Whatever your needs, Sitecore Content Hub makes it easy to create custom notifications. In this blog post, I'll show you how to create both realtime and email notifications using a little bit of scripting.
Realtime Notifications
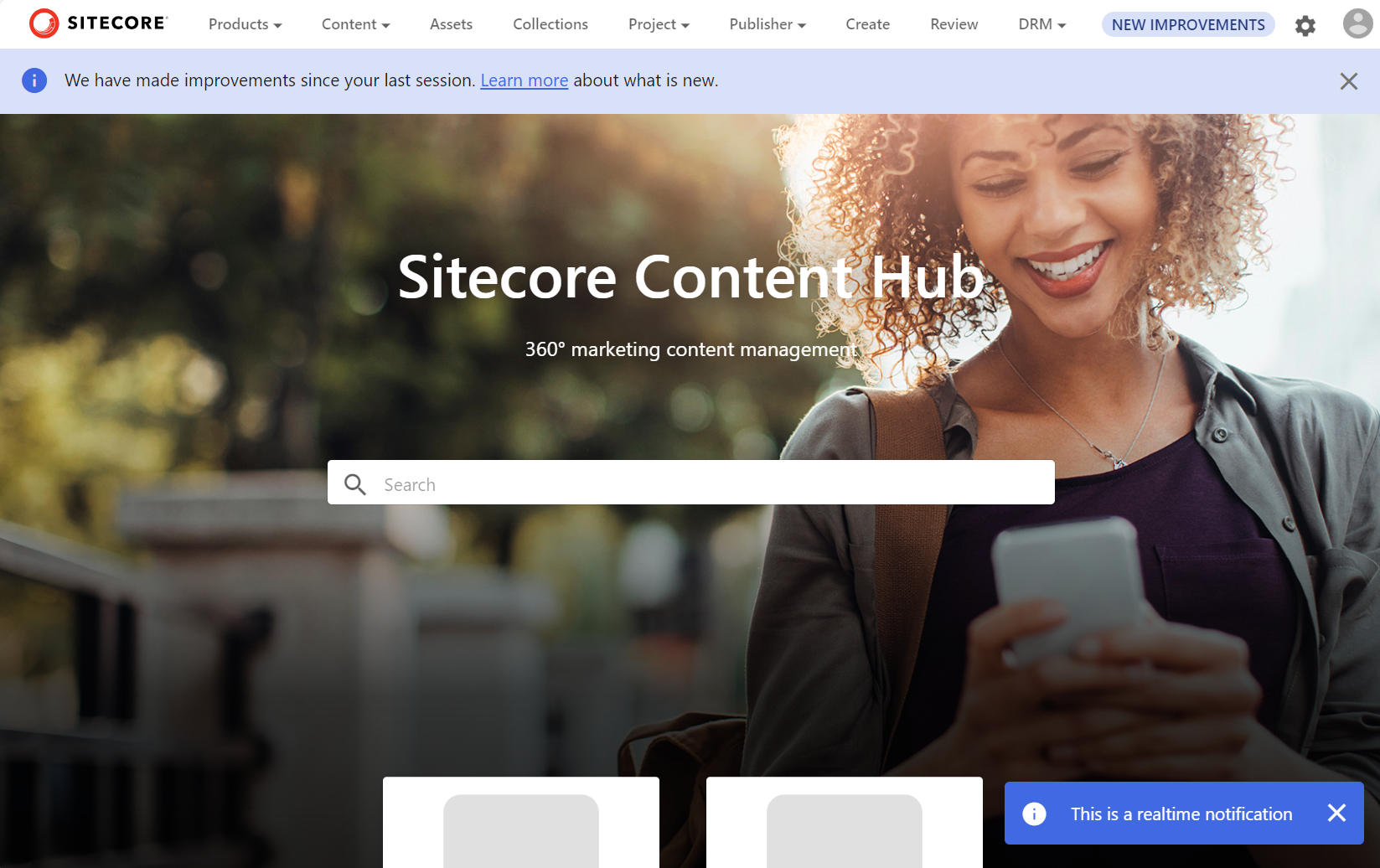
Realtime notifications are shown within the Content Hub UI, so they're a great way to get users' attention quickly. To send a realtime notification, you can use the SendRealTimeNotificationAsync
method.
Here's an example of a script that sends a realtime notification to the user nvn@sitecore.net:
using System.Collections.Generic;
using Stylelabs.M.Sdk.Models.Notifications;
var request = new RealtimeRequestByUsername
{
Title = "Visit Cat Island",
NotificationLevel = NotificationLevel.Info,
Link = new Uri("https://www.japan.travel/en/spot/1765"),
Recipients = new List<string>() { "nvn@sitecore.net" }
};
request.SetBody("It's happening - a new cat image has been uploaded!");
request.Validate();
await MClient.Notifications.SendRealTimeNotificationAsync(request);
Email Notifications
Email notifications are a good way to send more detailed information to users, or to reach users who aren't logged into Content Hub at the time. To send an email notification, you'll need to create an Email Template if one doesn't already exist.
Create An Email Template
To create an email template via the API, create a HTTP POST request to /api/entities
with the following body:
{
"entitydefinition": {
"href": "{{host}}/api/entitydefinitions/M.Mailing.Template"
},
"properties": {
"M.Mailing.TemplateName": "New Cat Notification",
"M.Mailing.TemplateLabel": {
"en-US": "New Cat Notification"
},
"M.Mailing.Subject": {
"en-US": "!! New Cat Alert !!"
},
"M.Mailing.Body": {
"en-US": "An awesome new cat image was uploaded on <i>{{Date}}</i>"
},
"M.Mailing.TemplateVariables": [
{
"Name": "Date",
"Type": "DateTime"
}
]
}
}
You will of course need to replace the {{host}}
variable, and perhaps the feline references. You can read more about email templates, including dynamic variables, in the Content Hub documentation.
Send an Email Notification
Once you have created an Email Template, you can send an email notification using the SendEmailNotificationAsync
method.
Here's an example of a script that sends an email notification to the user nvn@sitecore.net, using the Email Template we created previously:
using Newtonsoft.Json.Linq;
using System.Collections.Generic;
using Stylelabs.M.Sdk.Models.Notifications;
dynamic parameters = new JObject();
parameters.Date = DateTime.Now;
parameters.Culture = "en-US";
var request = new MailRequestByUsername
{
MailTemplateName = "New Cat Notification",
Variables = parameters,
Recipients = new List<string>() { "nvn@sitecore.net" }
};
request.Validate();
await MClient.Notifications.SendEmailNotificationAsync(request);
Additional Considerations
When implementing custom notifications, there are a few things you'll need to keep in mind:
- Trigger : How will the script be triggered? You could use a Trigger / Action combination within Content Hub, or execute it via the REST API.
- Business logic : What business logic should be executed within the script to either decided whether a notification should be sent, or to gather the data relevant for the content.
- Recipients : Who should the notification be delivered to?
- Notification Content : What should the content of the notification be, including, for email notifications, the email body and dynamic variables
#GoForIt
That's it! You now know how to create custom notifications in Sitecore Content Hub. So go forth and notify your users about all the latest and greatest cat content.
(And if you're feeling daring, you could even send a notification to everyone about a new cat image. Just don't blame me if you get fired. #IDareYou 😛)